
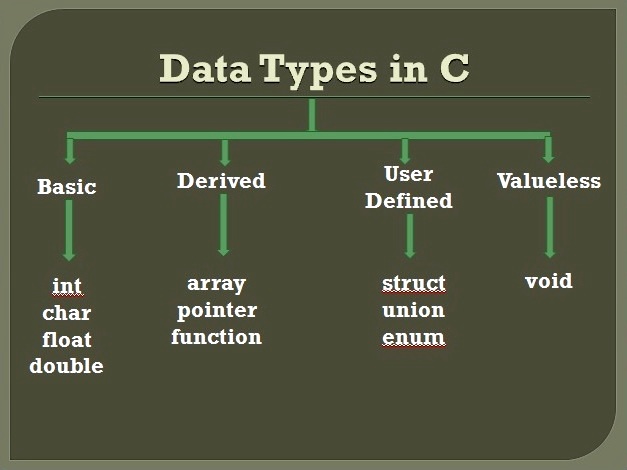
C stores the binary equivalent of the Unicode/ ASCII value of any character that we type. Just like the int data type, char can be signed (range from -128 to +127) or unsigned (0 to 255). Since its an array, we have to specify the length (30 in this case). Note that for a single character, we use single quotes, but for String (character array), we use double-quotes. Printf("group is %c, name is %s", group, name)
Basic data types in c programming language full#
To print a name or a full string, we need to define char array. Note the difference in outputs – while double prints the exact value, float value is rounded off to the nearest number. Long Double is treated the same as double by most compilers however, it was made for quadruple data precision. Float is just a single-precision data type double is the double-precision data type. This is useful in scientific programs that require precision. Double is 8 bytes, which means you can have more precision than float. You can think of float, double and long double similar to short int, int, and long int. The Data Science Course 2023: Complete Data Science Bootcamp Double Try to run this program and see what value you get. If you try to print the value of mark as %d after declaring it as float, you will not get 67. However, you will get the result of the mark as 67.00000, which may not be a pleasant sight with a lot of redundant zeroes.
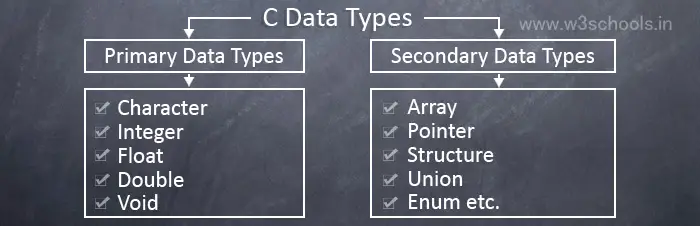
To print the exact value, we need ‘float’ data type.įloat is 4 bytes, and we can print the value using %f. if we use int data type, it will strip off the decimal part and print only 97. For example, average marks can be 97.665. The floating point data type allows the user to type decimal values. ‘short int’ can be used to limit the size of the integer data type. If we add more digits to short int num1 = 10000, it will be out of range and will print wrong value.
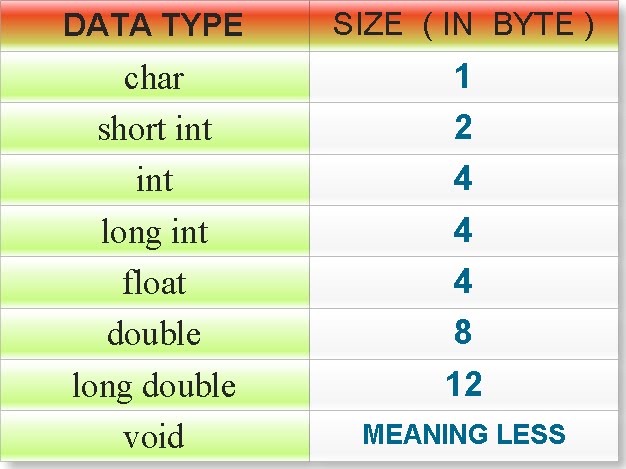
This is why it is safe to use %ld, unless you want the values to be always unsigned. Let us say the value of long notprime = -2300909090909933322 has a minus, but we print it as notprime is %lu, the correct value will not be printed. We can use %u in place of %d for unsigned int but even %d works. Though in practical situations, we may not use numbers that are this big, it is good to know the range and what data type we should use for programs with exponential calculations. Note that we have used ‘long long’ for sum, which is 8 bytes, whereas long is 4 bytes. We have used %hd for short, %d for int, and so on for printing each data type. Printf("num1 is % hd, number is % d, prime is % ld, notprime is % ld, sum is % lld", num1, number, prime, notprime, sum)
Basic data types in c programming language how to#
How to print integer variables? Here is a small program that you can try and tweak to get different results and understand the range of short, int, and long.
